Code testing in application development plays a vital role in ensuring the reliability, stability, and maintainability of software solutions. Essentially, there are many different types of code testing that can be performed on an application, including, but not limited to;
- Unit Testing
- Integration Testing
- System Testing
Each type of test is executed to achieve a specific goal in the Software Development Lifecycle (SDLC). This article outlines 3 of the main code testing practices and highlights the overall importance of incorporating testing practices throughout your SDLC.
Table of Contents
Ensuring reliability and quality
What is Code Testing?
Code testing refers to running each line of code with a controlled input, and verifying if it performs the expected output. The goal is to identify bugs or errors during the SDLC. While code documentation helps others to understand what you expect your code to do, testing guarantees that it meets these expectations.
Types of Code Testing
Unit Testing
Unit Testing is the process of testing individual units of code, such as functions or classes. For instance, in React, the common pattern is to divide the UI into smaller parts called “Components.” Writing Unit Tests for those components is a simple task since a component is normally responsible for just one thing: render a node in the DOM, which is great to do Unit Testing on. To demonstrate, let’s check the code below:
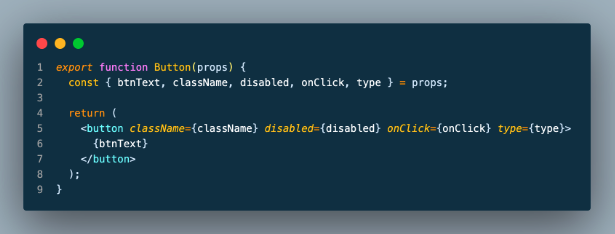
This example involves a simple button with no special behavior. However, it is important to note that if writing a Unit Test for a component in isolation is hard due to the need to satisfy a lot of dependencies (ie: Providers), it is a sign of a bad design but that is a topic for another post.
With that being said, let's write a Unit Test for this component:
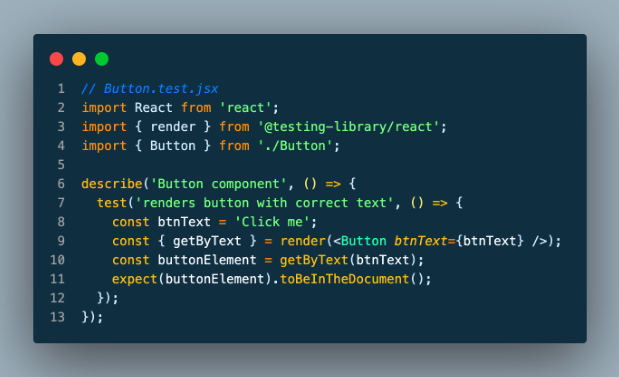
Essentially, this Unit Test is using testing-library/react and is “checking” that the button renders the text that we pass as a prop.
Similarly, you can continue writing Unit Tests to check every prop that you pass to the component. Those tests may not be outstanding, but at least they are checking that we need and use all the props and servers as a snapshot of the responsibilities of that specific component at that given time.
If in the future someone modifies the component to use a different prop for the text, this test will fail and provide an alert that something is not working as expected.
Integration Testing
Integration Testing refers to a type of Software Testing that focuses on verifying the interactions and communication between different components, modules, or subsystems of a software system. It aims to ensure that the integrated components work together as expected and that the overall system functions correctly.
The primary goal of this type of testing is to identify defects or issues that may arise when different components are combined and interact with each other. By testing the integration points and data flow between components, Integration Testing helps uncover errors such as:
- Incompatible interfaces
- Incorrect data transfers
- Communication failures
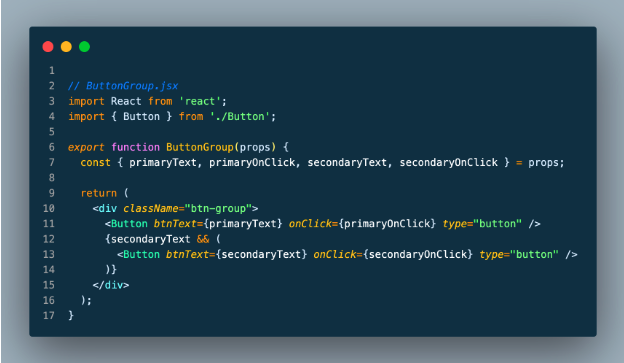
In this example, we took the first button component and created another slightly more complex one. The Integration Test for it could be as below:

Primarily, the first two tests of this code are more like Unit Tests, since they are simply checking that everything is working as expected. However, the third test marks the starting point for Integration Testing.
Basically, it checks that, if you don’t pass an argument for the secondary button, it will not be rendered. Consequently, it describes intent, behavior, and a spec that provides more details than a Unit Test.
It is important to remember that these tests work as the living documentation of the project, and from them, you can build the final user documentation from scratch.
End-to-End Testing
End-to-end Testing verifies that your software works correctly from the beginning to the end of a particular user flow. It replicates expected user behavior and various usage scenarios to ensure that your software works as a whole.
It uses a production-equivalent environment, as well as, data to simulate real-world situations, and may also involve the integrations your software has with external applications.
Writing this type of test requires more effort than the previous ones since, in this case, you need to take into account much more variables than before, such as:
- Is the user on the right path?
- Is the button disabled after X action?
- Is navigation blocked?
Typically, these types of variables are a little bit harder to debug.
With that being said, End-to-End Testing can be performed at various levels, including:
- Component Integration Testing: This level of software testing involves checking the interactions between individual components or modules on a page. It ensures that the components work together correctly and that they behave as expected.
- API Integration Testing: APIs enable different software systems to communicate and exchange data. API Integration Testing focuses on testing the integration and functionality of APIs, verifying that they correctly handle requests, process data, and return the expected responses.
- Database Integration Testing: In systems that rely on databases, Integration Testing may involve testing the interaction between the application and the database. It ensures that data is properly stored, retrieved, and updated, in addition to ensuring that the database schema aligns with the application's requirements.
- System Integration Testing: This level of Integration Testing examines the integration of all components and subsystems that make up the entire software system. It validates the end-to-end functionality, data flow, and interoperability of the system by simulating real-world scenarios and user interactions.
Another key point to note is that End-to-End Testing can be carried out either manually or automated using specialized testing frameworks and tools such as Cypress, Selenium, and Playwright, to name a few. These tools facilitate the creation of test cases that simulate interactions between pages/actions, in addition to automating the verification process.
Since End-to-End Testing verifies that all components of a system can run in real-world scenarios, the goal of this form of software testing is to simulate and validate the user experience (UX) from start to finish. The key here is the UX since those tests can catch any bug that any end user of your app could encounter beforehand.
As an example, let us assume that we have the following home page:
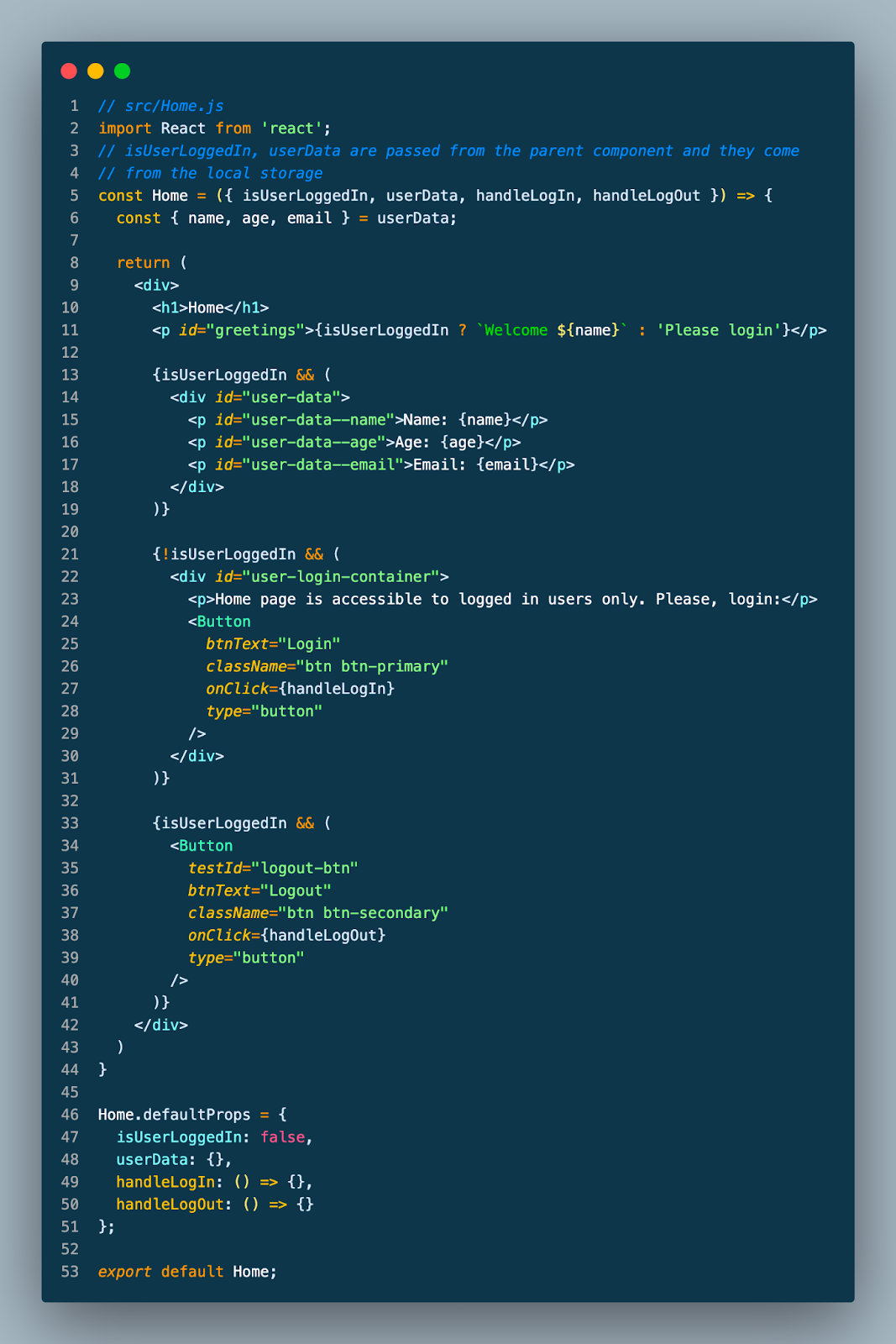
This is a simple home page that renders the name, age, and email of the logged user or renders a login button with a message that invites the user to log in.
Let's use Cypress to verify that the homepage works as intended:
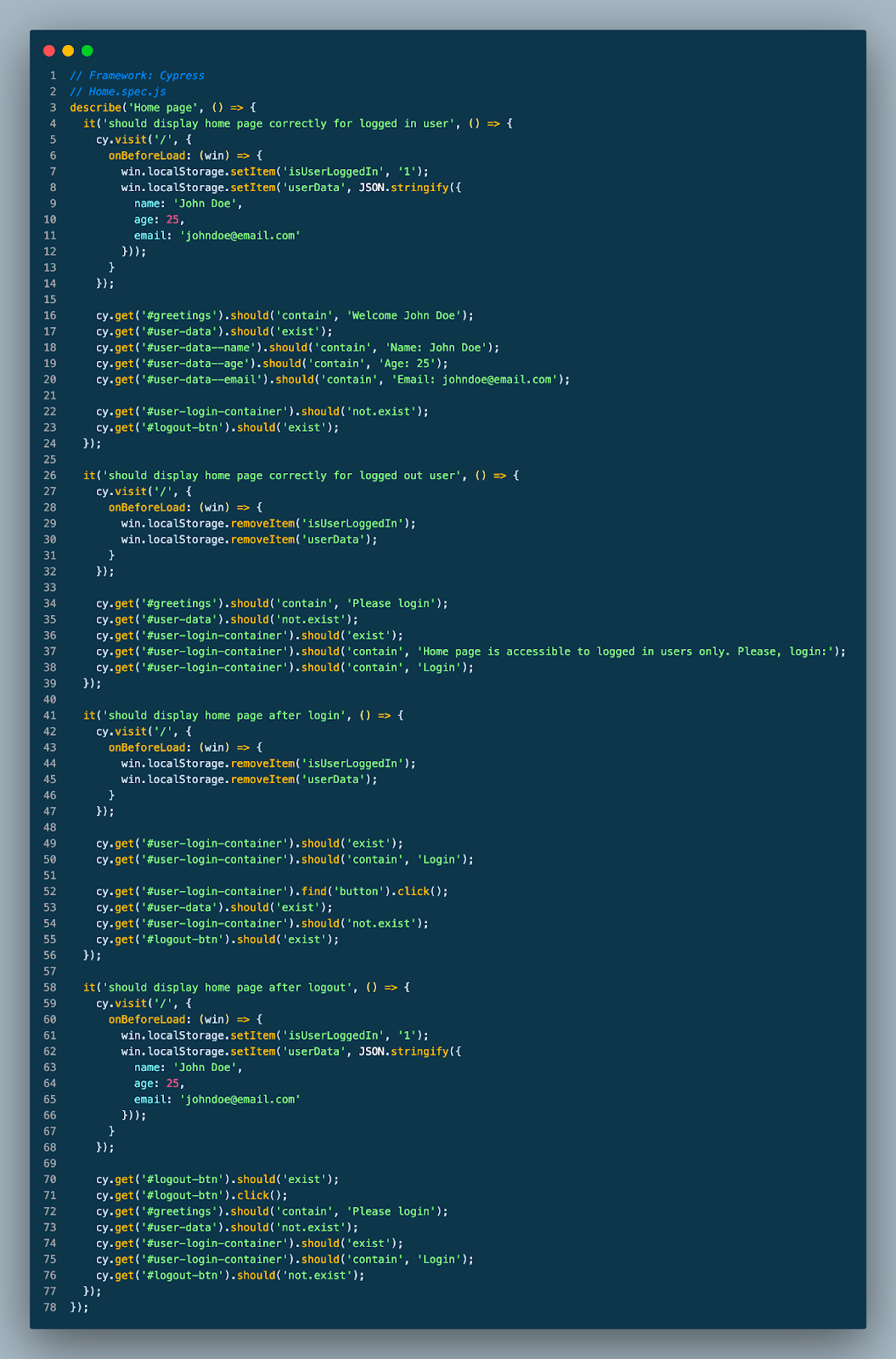
The first two Test Cases verify the logged-in and logged-out user states and describe the expected rendered output for each state.
The last two tests verify that the page renders the appropriate output after the user logs in or out. In essence, all four tests aim to ensure that the page renders correctly, but they do so in different ways.
The first two tests are somehow static and resemble the Unit Testing strategy (we can also apply that to End-to-End Tests) since they verify the rendered output without user interaction.
This is not “Bad” but, for those kinds of tests, we can use the Unit or Integration Testing. The last two tests are not “Perfect” but IMO they are more useful than the first ones and that's because they are dynamic.
Essentially, they verify the rendered output before and after the user has interacted with the page.
They also simulate the user interaction with our app, and in this case, we wrote some tests that verify the possible scenarios of the page.
If something is changed regarding the local storage or the way that the implementation of handleLogIn or handleLogOut works, this test will give an alert by failing.
Benefits of Code Testing
Ensuring Reliability and Quality
Code Testing serves as a shield that safeguards your applications from bugs, errors, and unforeseen issues. Its importance is not limited to guarding against bugs or errors since well-tested code can give you peace of mind on those long weekends or late Friday night deployments.
For this reason, by subjecting your code to a battery of tests you gain confidence in the reliability and quality of your software. The result is more shipping time and less time spent working on hotfixes.
Preventing Regression
As applications evolve and new features are added, it's vital to ensure that existing functionality remains intact. This is where software tests truly come in handy. Primarily, Regression Testing becomes essential to verify that changes in one part of the application do not inadvertently break other areas.
By employing comprehensive code testing, you can catch regression issues promptly, preventing a cascade of problems and saving valuable time that would otherwise be spent on debugging and troubleshooting.
In the absence of code testing, adding new functionality or refactoring existing code becomes an unnecessary, complex, and tedious task, since the Development and QA teams have to literally “test” each functionality of the app manually.
Enhancing Maintainability
Well-tested code is inherently more maintainable. When tests accompany your codebase, they serve as living documentation, allowing Developers to understand the expected behavior of different components. Consequently, by regularly running tests during Development, you can ensure that any changes or enhancements do not unintentionally alter existing functionality.
Moreover, testing helps in refactoring efforts by providing a safety net, since they give Developers the confidence to make necessary modifications while ensuring that everything still works as expected.
Facilitating Collaboration
Testing code promotes collaboration among Developers, Quality Assurance (QA) Engineers, and other project stakeholders. Essentially, when you write tests, they serve as a common language that enables you to communicate your intent and ensure that everyone is on the same page.
Furthermore, code tests help identify misunderstandings, align expectations, and serve as a tangible measure of progress. Additionally, QA engineers can focus on more comprehensive testing scenarios already aware that Developers have already covered the basics. This collaboration streamlines the Development process and enhances overall team productivity.
Building User Confidence
A well-tested application inspires trust and confidence in its users. This is down to the fact testing helps identify and fix issues that users might encounter, and in the process, provides a smoother and more enjoyable experience.
Moreover, by demonstrating a commitment to quality through comprehensive testing practices, you can establish a positive reputation, foster customer loyalty, and achieve user satisfaction.
Reducing Costs
While testing code requires an investment of time and resources upfront, it ultimately leads to cost savings in the long run. Early detection of defects reduces the effort and expenses associated with fixing them after deployment. Moreover, comprehensive code testing helps identify performance bottlenecks and scalability issues, enabling you to optimize your applications and avoid costly downtime in production environments.
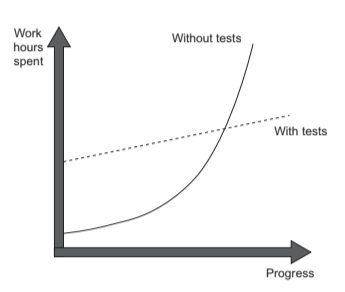
As we can see from the graphical representation above, in the absence of code tests, Development teams are forced to spend much more hours than necessary to make any meaningful progress. In contrast, with good tests the progression is linear. This aspect serves as the perfect summation of the other benefits of code testing that we have outlined.
But, what does “good tests” mean? or what makes a test “bad”? Primarily, while code testing can help projects grow, simply writing tests is not enough. Badly written code tests will still produce the same results. As shown below, bad code tests can help slow down code deterioration at the beginning, but they are not a long-term solution.
To maintain project growth, it is important to write well-designed code tests that can catch bugs and defects.

Conclusion
As we have seen, testing code is important for a number of reasons. It can help to identify and fix bugs early on in the Development process. By finding and fixing bugs, you can make the application more reliable and user-friendly while keeping the rest of the code safe. This can save a lot of time and money in the long run, as it is much easier to fix something that is not behaving as expected early on than it is to fix it after the application has been released.
As a Developer, you have a responsibility to deliver high-quality, reliable software solutions. Code testing is an indispensable tool in your arsenal that enables you to meet this objective. By incorporating software testing practices throughout the development process, you can ensure the reliability, stability, and maintainability of your applications, while building trust with users and minimizing the risk of costly defects at the same time.
Note: The screenshots were taken with the VS code plugin: Code Snap.
You may also be interested in:
Top Tips for Successful Nearshore Software Development
AWS vs Azure: Which is Better for Cloud Computing
Best DevOps Certifications: The Complete Guide for 2023
Nearshore Software Development Rates: The Complete Guide for 2023
More cost-effective than hiring in-house, with Nearshore Boost, our nearshore software development service, you can ensure your business stays competitive with an expanded team and a bigger global presence, you can be flexible as you respond to your customers’ needs.
Learn more about our services by booking a free consultation with us today!